Note
Go to the end to download the full example code.
Cueing Group Analysis Winter 2019
Setup
# Standard Pythonic imports
import os,sys,glob,numpy as np, pandas as pd
import scipy
from collections import OrderedDict
import warnings
warnings.filterwarnings('ignore')
from matplotlib import pyplot as plt
import matplotlib.patches as patches
# MNE functions
from mne import Epochs, find_events, concatenate_raws
from mne.time_frequency import tfr_morlet
# EEG-Noteooks functions
from eegnb.analysis.analysis_utils import load_data
from eegnb.datasets import fetch_dataset
Load the data
eegnb_data_path = os.path.join(os.path.expanduser('~/'),'.eegnb', 'data')
cueing_data_path = os.path.join(eegnb_data_path, 'visual-cueing', 'kylemathlab_dev')
# If dataset hasn't been downloaded yet, download it
if not os.path.isdir(cueing_data_path):
fetch_dataset(data_dir=eegnb_data_path, experiment='visual-cueing', site='kylemathlab_dev')
Put the data into MNE Epochs
Fall 2018 subs = [101, 102, 103, 104, 105, 106, 108, 109, 110, 111, 112,
202, 203, 204, 205, 207, 208, 209, 210, 211, 301, 302, 303, 304, 305, 306, 307, 308, 309]
Winter 2019
subs = [1101, 1102, 1103, 1104, 1105, 1106, 1108, 1109, 1110,
1202, 1203, 1205, 1206, 1209, 1210, 1211, 1215,
1301, 1302, 1313,
1401, 1402, 1403, 1404, 1405, 1408, 1410, 1411, 1412, 1413, 1413, 1414, 1415, 1416]
#
# Both
# subs = [101, 102, 103, 104, 105, 106, 108, 109, 110, 111, 112,
# 202, 203, 204, 205, 207, 208, 209, 210, 211,
# 301, 302, 303, 304, 305, 306, 307, 308, 309,
# 1101, 1102, 1103, 1104, 1105, 1106, 1108, 1109, 1110,
# 1202, 1203, 1205, 1206, 1209, 1210, 1211, 1215,
# 1301, 1302, 1313,
# 1401, 1402, 1403, 1404, 1405, 1408, 1410, 1411, 1412, 1413, 1413, 1414, 1415, 1416]
#
#
# placeholders to add to for each subject
diff_out = []
Ipsi_out = []
Contra_out = []
Ipsi_spectra_out = []
Contra_spectra_out = []
diff_spectra_out = []
ERSP_diff_out = []
ERSP_Ipsi_out = []
ERSP_Contra_out = []
frequencies = np.linspace(6, 30, 100, endpoint=True)
wave_cycles = 6
# time frequency window for analysis
f_low = 7 # Hz
f_high = 10
f_diff = f_high-f_low
t_low = 0 # s
t_high = 1
t_diff = t_high-t_low
bad_subs= [6, 7, 13, 26]
really_bad_subs = [11, 12, 19]
sub_count = 0
for sub in subs:
print(sub)
sub_count += 1
if (sub_count in really_bad_subs):
rej_thresh_uV = 90
elif (sub_count in bad_subs):
rej_thresh_uV = 90
else:
rej_thresh_uV = 90
rej_thresh = rej_thresh_uV*1e-6
# Load both sessions
raw = load_data(sub,1, # subject, session
experiment='visual-cueing',site='kylemathlab_dev',device_name='muse2016',
data_dir = eegnb_data_path)
raw.append(
load_data(sub,2, # subject, session
experiment='visual-cueing', site='kylemathlab_dev', device_name='muse2016',
data_dir = eegnb_data_path))
# Filter Raw Data
raw.filter(1,30, method='iir')
#Select Events
events = find_events(raw)
event_id = {'LeftCue': 1, 'RightCue': 2}
epochs = Epochs(raw, events=events, event_id=event_id,
tmin=-1, tmax=2, baseline=(-1, 0),
reject={'eeg':rej_thresh}, preload=True,
verbose=False, picks=[0, 3])
print('Trials Remaining: ' + str(len(epochs.events)) + '.')
# Compute morlet wavelet
# Left Cue
tfr, itc = tfr_morlet(epochs['LeftCue'], freqs=frequencies,
n_cycles=wave_cycles, return_itc=True)
tfr = tfr.apply_baseline((-1,-.5),mode='mean')
power_Ipsi_TP9 = tfr.data[0,:,:]
power_Contra_TP10 = tfr.data[1,:,:]
# Right Cue
tfr, itc = tfr_morlet(epochs['RightCue'], freqs=frequencies,
n_cycles=wave_cycles, return_itc=True)
tfr = tfr.apply_baseline((-1,-.5),mode='mean')
power_Contra_TP9 = tfr.data[0,:,:]
power_Ipsi_TP10 = tfr.data[1,:,:]
# Compute averages Differences
power_Avg_Ipsi = (power_Ipsi_TP9+power_Ipsi_TP10)/2;
power_Avg_Contra = (power_Contra_TP9+power_Contra_TP10)/2;
power_Avg_Diff = power_Avg_Ipsi-power_Avg_Contra;
#output data into array
times = epochs.times
Ipsi_out.append(np.mean(power_Avg_Ipsi[np.argmax(frequencies>f_low):
np.argmax(frequencies>f_high)-1,
np.argmax(times>t_low):np.argmax(times>t_high)-1 ]
)
)
Ipsi_spectra_out.append(np.mean(power_Avg_Ipsi[:,np.argmax(times>t_low):
np.argmax(times>t_high)-1 ],1
)
)
Contra_out.append(np.mean(power_Avg_Contra[np.argmax(frequencies>f_low):
np.argmax(frequencies>f_high)-1,
np.argmax(times>t_low):np.argmax(times>t_high)-1 ]
)
)
Contra_spectra_out.append(np.mean(power_Avg_Contra[:,np.argmax(times>t_low):
np.argmax(times>t_high)-1 ],1))
diff_out.append(np.mean(power_Avg_Diff[np.argmax(frequencies>f_low):
np.argmax(frequencies>f_high)-1,
np.argmax(times>t_low):np.argmax(times>t_high)-1 ]
)
)
diff_spectra_out.append(np.mean(power_Avg_Diff[:,np.argmax(times>t_low):
np.argmax(times>t_high)-1 ],1
)
)
#save the spectrograms to average over after
ERSP_diff_out.append(power_Avg_Diff)
ERSP_Ipsi_out.append(power_Avg_Ipsi)
ERSP_Contra_out.append(power_Avg_Contra)
1101
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1101/session001/subject1101_session1_recording_2019-03-26-20.08.20.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1101/session002/subject1101_session2_recording_2019-03-26-20.16.25.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
67 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 1.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1102
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1102/session001/subject1102_session1_recording_2019-03-26-20.27.26.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1102/session002/subject1102_session2_recording_2019-03-26-20.35.07.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
136 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 31.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1103
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1103/session001/subject1103_session1_recording_2019-03-26-20.45.55.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1103/session002/subject1103_session2_recording_2019-03-26-20.52.46.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
161 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 64.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1104
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1104/session001/subject1104_session1_recording_2019-03-26-21.02.02.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1104/session002/subject1104_session2_recording_2019-03-26-21.08.38.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
187 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 51.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1105
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1105/session001/subject1105_session1_recording_2019-03-26-21.18.06.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1105/session002/subject1105_session2_recording_2019-03-26-21.24.15.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
192 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 73.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1106
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1106/session001/subject1106_session1_recording_2019-03-26-21.37.04.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1106/session002/subject1106_session2_recording_2019-03-26-21.44.54.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
134 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 56.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1108
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1108/session001/subject1108_session1_recording_2019-03-28-20.37.17.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1108/session002/subject1108_session2_recording_2019-03-28-20.44.39.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
146 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 25.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1109
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1109/session001/subject1109_session1_recording_2019-03-28-20.53.09.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1109/session002/subject1109_session2_recording_2019-03-28-21.00.47.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
176 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 47.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1110
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1110/session001/subject1110_session1_recording_2019-03-28-21.09.52.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1110/session002/subject1110_session2_recording_2019-03-28-21.16.24.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
168 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 12.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1202
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1202/session001/subject1202_session1_recording_2019-03-26-21.00.19.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61308
Range : 0 ... 61307 = 0.000 ... 239.480 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1202/session002/subject1202_session2_recording_2019-03-26-21.07.19.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
173 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 73.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1203
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1203/session001/subject1203_session1_recording_2019-03-26-21.18.01.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1203/session002/subject1203_session2_recording_2019-03-26-21.23.52.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
211 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 88.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1205
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1205/session001/subject1205_session1_recording_2019-03-26-20.27.40.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61308
Range : 0 ... 61307 = 0.000 ... 239.480 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1205/session002/subject1205_session2_recording_2019-03-26-20.33.10.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61308
Range : 0 ... 61307 = 0.000 ... 239.480 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
226 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 83.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1206
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1206/session001/subject1206_session1_recording_2019-03-26-20.43.14.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61308
Range : 0 ... 61307 = 0.000 ... 239.480 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1206/session002/subject1206_session2_recording_2019-03-26-20.49.14.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
214 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 66.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1209
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1209/session001/subject1209_session1_recording_2019-03-28-20.35.06.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1209/session002/subject1209_session2_recording_2019-03-28-20.41.47.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
186 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 40.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1210
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1210/session001/subject1210_session1_recording_2019-03-28-20.51.18.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1210/session002/subject1210_session2_recording_2019-03-28-20.57.32.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
198 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 27.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1211
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1211/session001/subject1211_session1_recording_2019-03-28-20.13.27.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1211/session002/subject1211_session2_recording_2019-03-28-20.23.34.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
139 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 53.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1215
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1215/session001/subject1215_session1_recording_2019-03-28-21.07.04.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1215/session002/subject1215_session2_recording_2019-03-28-21.13.37.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
193 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 46.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1301
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1301/session001/subject1301_session1_recording_2019-03-26-20.10.43.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1301/session002/subject1301_session2_recording_2019-03-26-20.17.14.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
197 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 0.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1302
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1302/session001/subject1302_session1_recording_2019-03-26-20.30.19.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1302/session002/subject1302_session2_recording_2019-03-26-20.36.50.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
194 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 0.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1313
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1313/session001/subject1313_session1_recording_2019-03-28-21.32.56.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1313/session002/subject1313_session2_recording_2019-03-28-21.39.30.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
186 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 56.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1401
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1401/session001/subject1401_session1_recording_2019-03-26-20.03.18.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1401/session002/subject1401_session2_recording_2019-03-26-20.11.54.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
125 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 43.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1402
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1402/session001/subject1402_session1_recording_2019-03-26-20.23.18.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1402/session002/subject1402_session2_recording_2019-03-26-20.31.17.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
158 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 65.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1403
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1403/session001/subject1403_session1_recording_2019-03-26-20.40.55.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1403/session002/subject1403_session2_recording_2019-03-26-20.48.07.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
172 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 17.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1404
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1404/session001/subject1404_session1_recording_2019-03-26-20.58.09.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1404/session002/subject1404_session2_recording_2019-03-26-21.06.17.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
112 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 38.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1405
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1405/session001/subject1405_session1_recording_2019-03-26-21.17.49.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1405/session002/subject1405_session2_recording_2019-03-26-21.25.03.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
162 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 26.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1408
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1408/session001/subject1408_session1_recording_2019-03-26-21.36.18.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1408/session002/subject1408_session2_recording_2019-03-26-21.44.04.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61284
Range : 0 ... 61283 = 0.000 ... 239.387 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
171 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 16.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1410
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1410/session001/subject1410_session1_recording_2019-03-28-20.01.52.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61308
Range : 0 ... 61307 = 0.000 ... 239.480 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1410/session002/subject1410_session2_recording_2019-03-28-20.09.14.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
172 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 53.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1411
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1411/session001/subject1411_session1_recording_2019-03-28-21.24.26.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1411/session002/subject1411_session2_recording_2019-03-28-21.31.09.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
160 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 53.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1412
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1412/session001/subject1412_session1_recording_2019-03-28-21.09.06.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1412/session002/subject1412_session2_recording_2019-03-28-21.15.30.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
168 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 46.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1413
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1413/session001/subject1413_session1_recording_2019-03-28-20.34.09.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1413/session002/subject1413_session2_recording_2019-03-28-20.40.57.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
154 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 41.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1413
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1413/session001/subject1413_session1_recording_2019-03-28-20.34.09.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1413/session002/subject1413_session2_recording_2019-03-28-20.40.57.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
154 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 41.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1414
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1414/session001/subject1414_session1_recording_2019-03-28-20.18.34.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61308
Range : 0 ... 61307 = 0.000 ... 239.480 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1414/session002/subject1414_session2_recording_2019-03-28-20.24.57.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
185 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 81.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1415
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1415/session001/subject1415_session1_recording_2019-03-28-21.39.58.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1415/session002/subject1415_session2_recording_2019-03-28-21.47.11.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
158 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 19.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
1416
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1416/session001/subject1416_session1_recording_2019-03-28-20.51.40.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Loading these files:
/home/runner/.eegnb/data/visual-cueing/kylemathlab_dev/muse2016/subject1416/session002/subject1416_session2_recording_2019-03-28-20.58.50.csv
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
['TP9', 'AF7', 'AF8', 'TP10', 'Right AUX', 'stim']
Creating RawArray with float64 data, n_channels=6, n_times=61296
Range : 0 ... 61295 = 0.000 ... 239.434 secs
Ready.
Filtering raw data in 2 contiguous segments
Setting up band-pass filter from 1 - 30 Hz
IIR filter parameters
---------------------
Butterworth bandpass zero-phase (two-pass forward and reverse) non-causal filter:
- Filter order 16 (effective, after forward-backward)
- Cutoffs at 1.00, 30.00 Hz: -6.02, -6.02 dB
160 events found on stim channel stim
Event IDs: [ 1 2 11 12 21 22]
Trials Remaining: 40.
Applying baseline correction (mode: mean)
Applying baseline correction (mode: mean)
Combine subjects
#average spectrograms
GrandAvg_diff = np.nanmean(ERSP_diff_out,0)
GrandAvg_Ipsi = np.nanmean(ERSP_Ipsi_out,0)
GrandAvg_Contra = np.nanmean(ERSP_Contra_out,0)
#average spectra
GrandAvg_spec_Ipsi = np.nanmean(Ipsi_spectra_out,0)
GrandAvg_spec_Contra = np.nanmean(Contra_spectra_out,0)
GrandAvg_spec_diff = np.nanmean(diff_spectra_out,0)
#error bars for spectra (standard error)
num_good = len(diff_out) - sum(np.isnan(diff_out))
GrandAvg_spec_Ipsi_ste = np.nanstd(Ipsi_spectra_out,0)/np.sqrt(num_good)
GrandAvg_spec_Contra_ste = np.nanstd(Contra_spectra_out,0)/np.sqrt(num_good)
GrandAvg_spec_diff_ste = np.nanstd(diff_spectra_out,0)/np.sqrt(num_good)
#Plot Spectra error bars
fig, ax = plt.subplots(1)
plt.errorbar(frequencies,GrandAvg_spec_Ipsi,yerr=GrandAvg_spec_Ipsi_ste)
plt.errorbar(frequencies,GrandAvg_spec_Contra,yerr=GrandAvg_spec_Contra_ste)
plt.legend(('Ipsi','Contra'))
plt.xlabel('Frequency (Hz)')
plt.ylabel('Power (uV^2)')
plt.hlines(0,3,33)
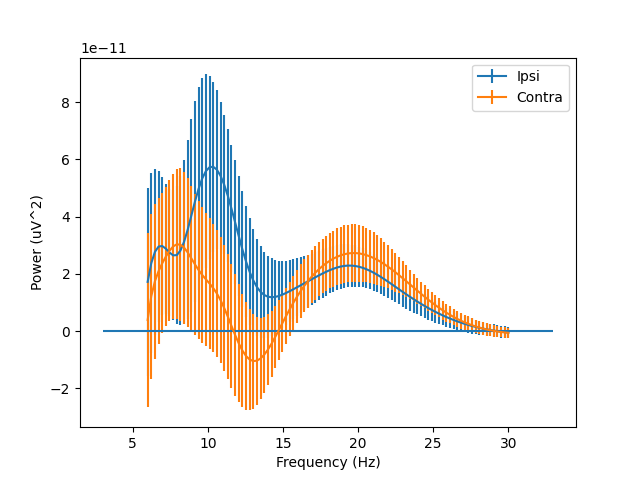
<matplotlib.collections.LineCollection object at 0x7fa89dd72280>
#Plot Spectra Diff error bars
fig, ax = plt.subplots(1)
plt.errorbar(frequencies,GrandAvg_spec_diff,yerr=GrandAvg_spec_diff_ste)
plt.legend('Ipsi-Contra')
plt.xlabel('Frequency (Hz)')
plt.ylabel('Power (uV^2)')
plt.hlines(0,3,33)
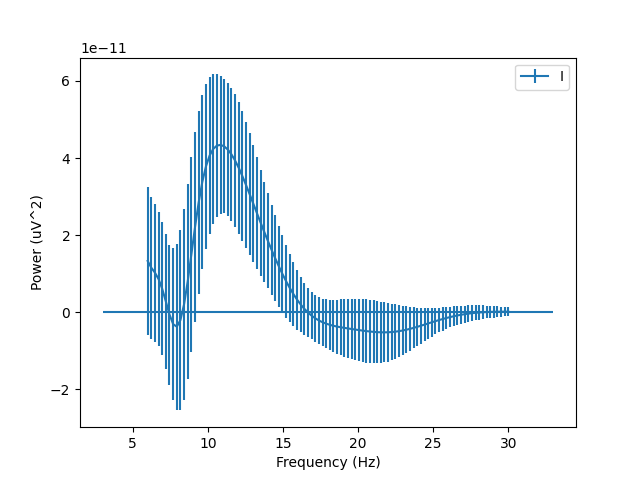
<matplotlib.collections.LineCollection object at 0x7fa89df31f10>
Grand Average Ipsi
plot_max = np.max([np.max(np.abs(GrandAvg_Ipsi)), np.max(np.abs(GrandAvg_Contra))])
fig, ax = plt.subplots(1)
im = plt.imshow(GrandAvg_Ipsi,
extent=[times[0], times[-1], frequencies[0], frequencies[-1]],
aspect='auto', origin='lower', cmap='coolwarm', vmin=-plot_max, vmax=plot_max)
plt.xlabel('Time (sec)')
plt.ylabel('Frequency (Hz)')
plt.title('Power Ipsi')
cb = fig.colorbar(im)
cb.set_label('Power')
# Create a Rectangle patch
rect = patches.Rectangle((t_low,f_low),t_diff,f_diff,linewidth=1,edgecolor='k',facecolor='none')
# Add the patch to the Axes
ax.add_patch(rect)
#
##e#################################################################################################
#
# Grand Average Contra
#
fig, ax = plt.subplots(1)
im = plt.imshow(GrandAvg_Contra,
extent=[times[0], times[-1], frequencies[0], frequencies[-1]],
aspect='auto', origin='lower', cmap='coolwarm', vmin=-plot_max, vmax=plot_max)
plt.xlabel('Time (sec)')
plt.ylabel('Frequency (Hz)')
plt.title('Power Contra')
cb = fig.colorbar(im)
cb.set_label('Power')
# Create a Rectangle patch
rect = patches.Rectangle((t_low,f_low),t_diff,f_diff,linewidth=1,edgecolor='k',facecolor='none')
# Add the patch to the Axes
ax.add_patch(rect)
#
<matplotlib.patches.Rectangle object at 0x7fa89e079550>
Grand Average Ipsi-Contra Difference
plot_max_diff = np.max(np.abs(GrandAvg_diff))
fig, ax = plt.subplots(1)
im = plt.imshow(GrandAvg_diff,
extent=[times[0], times[-1], frequencies[0], frequencies[-1]],
aspect='auto', origin='lower', cmap='coolwarm', vmin=-plot_max_diff, vmax=plot_max_diff)
plt.xlabel('Time (sec)')
plt.ylabel('Frequency (Hz)')
plt.title('Power Difference Ipsi-Contra')
cb = fig.colorbar(im)
cb.set_label('Ipsi-Contra Power')
# Create a Rectangle patch
rect = patches.Rectangle((t_low,f_low),t_diff,f_diff,linewidth=1,edgecolor='k',facecolor='none')
# Add the patch to the Axes
ax.add_patch(rect)
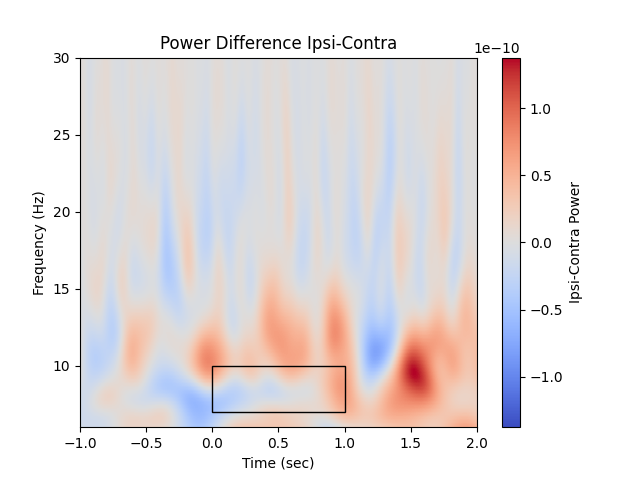
<matplotlib.patches.Rectangle object at 0x7fa89e0e5cd0>
Compute t test
num_good = len(diff_out) - sum(np.isnan(diff_out))
[tstat, pval] = scipy.stats.ttest_ind(diff_out,np.zeros(len(diff_out)),nan_policy='omit')
print('Ipsi Mean: '+ str(np.nanmean(Ipsi_out)))
print('Contra Mean: '+ str(np.nanmean(Contra_out)))
print('Mean Diff: '+ str(np.nanmean(diff_out)))
print('t(' + str(num_good-1) + ') = ' + str(round(tstat,3)))
print('p = ' + str(round(pval,3)))
Ipsi Mean: 3.5735930197157026e-11
Contra Mean: 2.6421745679207323e-11
Mean Diff: 9.314184517949713e-12
t(30) = 0.456
p = 0.65
Save average powers ipsi and contra
print(diff_out)
raw_data = {'Ipsi Power': Ipsi_out,
'Contra Power': Contra_out}
df = pd.DataFrame(raw_data, columns = ['Ipsi Power', 'Contra Power'])
print(df)
df.to_csv('375CueingEEG.csv')
print('Saved subject averages for each condition to 375CueingEEG.csv file in present directory')
[nan, 1.789004769369718e-11, 6.402571924638044e-11, 1.201698855806472e-10, 1.6436811298970808e-10, 1.1465401250727375e-10, 8.107123680349165e-11, -3.1169419915324295e-11, 6.49372710835747e-11, 4.3529314385416065e-11, 2.8760356184521736e-11, 3.773350340044497e-11, 2.113134995947907e-11, 1.5571207459949488e-10, -4.6291150675500723e-10, -8.052232335321791e-11, -4.6644221372822607e-11, nan, nan, -3.438951097875039e-11, 7.091042185483073e-11, 1.298338355801429e-11, 1.3604198590381474e-10, -5.861633427481533e-11, -6.204912953285817e-11, 1.8344086913139518e-10, 2.380242500968444e-11, -8.805033297719282e-11, -7.508128947253835e-11, 2.907964859843707e-11, 2.907964859843707e-11, -4.146716305783299e-11, -1.5307351374032941e-10, 2.3393198398387354e-11]
Ipsi Power Contra Power
0 NaN NaN
1 -3.901325e-11 -5.690330e-11
2 4.568905e-11 -1.833667e-11
3 2.037251e-11 -9.979737e-11
4 2.567893e-10 9.242117e-11
5 1.128359e-10 -1.818109e-12
6 3.090019e-10 2.279306e-10
7 -1.325457e-10 -1.013762e-10
8 4.086011e-11 -2.407716e-11
9 8.168927e-11 3.815995e-11
10 1.073335e-10 7.857313e-11
11 5.134699e-10 4.757364e-10
12 -1.088382e-11 -3.201517e-11
13 1.006811e-10 -5.503096e-11
14 -2.543183e-10 2.085932e-10
15 -3.615777e-11 4.436455e-11
16 -5.354581e-11 -6.901593e-12
17 NaN NaN
18 NaN NaN
19 5.637907e-13 3.495330e-11
20 -9.265134e-12 -8.017556e-11
21 -2.448927e-10 -2.578761e-10
22 2.682534e-10 1.322114e-10
23 4.775386e-11 1.063702e-10
24 -5.500639e-11 7.042736e-12
25 1.907671e-11 -1.643642e-10
26 2.746872e-11 3.666293e-12
27 -9.699470e-11 -8.944371e-12
28 1.345766e-10 2.096579e-10
29 3.654300e-11 7.463347e-12
30 3.654300e-11 7.463347e-12
31 -3.302737e-11 8.439791e-12
32 -4.426000e-11 1.088135e-10
33 -4.177686e-11 -6.517005e-11
Saved subject averages for each condition to 375CueingEEG.csv file in present directory
Save spectra
df = pd.DataFrame(Ipsi_spectra_out,columns=frequencies)
print(df)
df.to_csv('375CueingIpsiSpec.csv')
df = pd.DataFrame(Contra_spectra_out,columns=frequencies)
df.to_csv('375CueingContraSpec.csv')
print('Saved Spectra to 375CueingContraSpec.csv file in present directory')
6.000000 6.242424 ... 29.757576 30.000000
0 NaN NaN ... NaN NaN
1 7.991890e-11 8.036284e-11 ... -2.094144e-11 -2.057510e-11
2 8.482874e-11 8.635330e-11 ... -3.867214e-12 -3.719235e-12
3 1.394826e-10 1.324403e-10 ... -3.411441e-12 -4.411093e-12
4 1.355015e-10 1.519385e-10 ... -5.270396e-12 -5.232011e-12
5 4.146935e-11 4.747567e-11 ... -6.757861e-12 -6.344323e-12
6 1.591767e-10 1.802644e-10 ... -3.011956e-12 -3.111111e-12
7 2.051924e-10 1.359738e-10 ... 9.878931e-12 9.096915e-12
8 -2.289620e-11 -1.966269e-11 ... -5.976043e-12 -5.762940e-12
9 2.639212e-10 2.390503e-10 ... 1.273891e-12 1.085691e-12
10 1.734091e-10 1.751927e-10 ... 1.127110e-12 1.042786e-12
11 -4.155194e-11 5.554974e-11 ... 3.215457e-11 3.061286e-11
12 1.132429e-10 9.957683e-11 ... -7.976622e-13 -6.777715e-13
13 1.521996e-10 2.196098e-10 ... -1.447362e-11 -1.356378e-11
14 -1.992109e-12 8.659712e-12 ... 1.917412e-12 1.278172e-12
15 -1.632265e-10 -1.465498e-10 ... -4.835722e-12 -4.643791e-12
16 1.051757e-10 9.010762e-11 ... 5.687247e-12 5.318740e-12
17 NaN NaN ... NaN NaN
18 NaN NaN ... NaN NaN
19 -3.037587e-11 -3.195487e-11 ... -9.292653e-13 -1.176655e-12
20 -4.576869e-11 -7.575594e-11 ... 5.139473e-12 4.728775e-12
21 -3.017372e-10 -2.806035e-10 ... -1.168381e-13 -2.039849e-13
22 1.892637e-10 1.928711e-10 ... -1.549501e-12 -1.594952e-12
23 -1.863042e-10 -1.441354e-10 ... 9.789338e-12 9.213076e-12
24 -1.099290e-10 -1.131996e-10 ... 6.197682e-12 5.769963e-12
25 -2.486010e-10 -1.910190e-10 ... 9.456576e-12 9.193358e-12
26 -2.444701e-10 -2.199233e-10 ... 1.492537e-11 1.519870e-11
27 -1.015551e-10 -9.978091e-11 ... -1.160645e-11 -1.092205e-11
28 -1.236814e-10 -1.335374e-10 ... 2.298211e-12 2.066240e-12
29 -1.196307e-10 -1.035983e-10 ... 3.293275e-12 2.487449e-12
30 -1.196307e-10 -1.035983e-10 ... 3.293275e-12 2.487449e-12
31 4.570382e-11 2.986854e-11 ... 2.506011e-12 2.287051e-12
32 6.194128e-10 6.055475e-10 ... -1.599190e-12 -1.352438e-12
33 -1.126135e-10 -1.308744e-10 ... -3.366325e-11 -3.285204e-11
[34 rows x 100 columns]
Saved Spectra to 375CueingContraSpec.csv file in present directory
Total running time of the script: (0 minutes 24.537 seconds)